Amazon Web Services (AWS) Command Line Interface (CLI) provides a set of commands for managing various AWS services, including EC2 instances. In order to use AWS CLI, you should have the AWS CLI installed and configured with the necessary credentials to run these commands successfully.
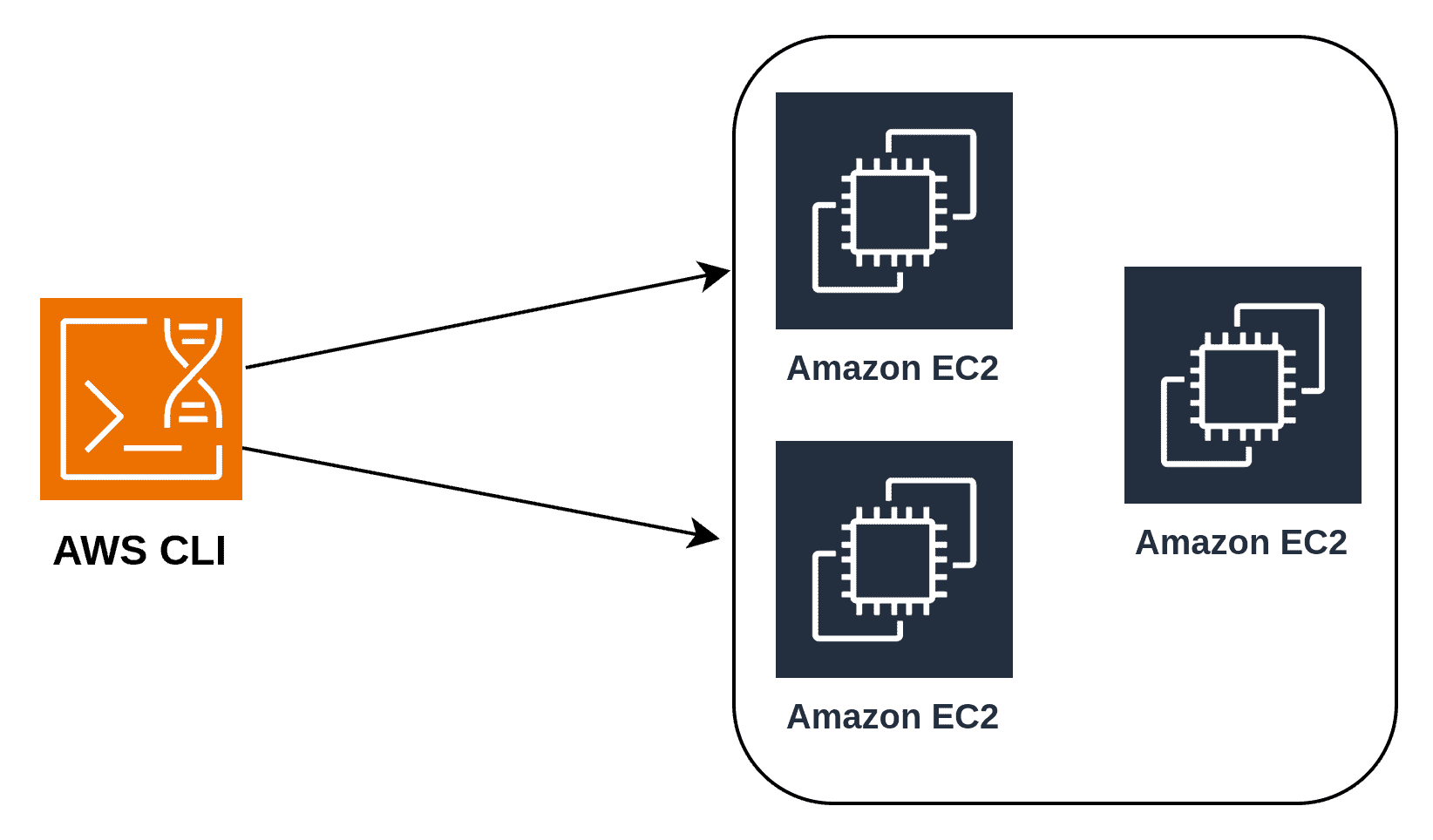
Here are some common AWS CLI commands for managing EC2 instances:
Create EC2 Instance:
To create an EC2 instance using the AWS CLI, you can use the AWS EC2 run-instances command. Below is an example command with some common parameters. Make sure to replace placeholder values (such as –image-id, –instance-type, and –key-name) with your actual values.
aws ec2 run-instances \
–image-id ami-xxxxxxxxxxxxxxxxx \
–instance-type t2.micro \
–key-name YourKeyPair \
–subnet-id subnet-xxxxxxxxxxxxxxxxx \
–security-group-ids sg-xxxxxxxxxxxxxxxxx \
–tag-specifications ‘ResourceType=instance,Tags=[{Key=Name,Value=YourInstanceName}]’
Explanation of parameters:
–image-id: The ID of the Amazon Machine Image (AMI) you want to use.
–instance-type: The type of EC2 instance you want to launch (e.g., t2.micro).
–key-name: The name of the key pair you created to access the instance.
–subnet-id: The ID of the subnet in which to launch the instance.
–security-group-ids: The security group(s) to associate with the instance.
–tag-specifications: Tags help you identify and organize your instances. In this example, a “Name” tag is added to the instance.
List EC2 Instances:
To list EC2 instances using the AWS CLI, you can use the AWS EC2 describe-instances command. Below is the basic command:
aws ec2 describe-instances
This command provides detailed information about all EC2 instances in your AWS account, including their current state, instance IDs, IP addresses, and more.
If you want to filter the output to display specific information or a subset of instances, you can use the –query parameter. For example, to display only the instance IDs and public IP addresses, you can use:
aws ec2 describe-instances –query ‘Reservations[*].Instances[*].[InstanceId, PublicIpAddress]’ –output table
Adjust the –query parameter based on the specific information you are interested in.
Start an EC2 Instance:
To start an EC2 instance using the AWS CLI, you can use the AWS EC2 start-instances command. Below is the basic command:
aws ec2 start-instances –instance-ids YourInstanceId
Replace YourInstanceId with the actual ID of the EC2 instance you want to start.
Here’s an example:
aws ec2 start-instances –instance-ids i-0abcdef1234567890
If successful, the command will output information about the instance state change. You can verify the status by using the AWS EC2 describe-instances command.
Stop an EC2 Instance:
To stop an EC2 instance using the AWS CLI, you can use the AWS EC2 stop-instances command. Below is the basic command:
aws ec2 stop-instances –instance-ids YourInstanceId
Replace YourInstanceId with the actual ID of the EC2 instance you want to stop.
Here’s an example:
aws ec2 stop-instances –instance-ids i-0abcdef1234567890
If successful, the command will output information about the instance state change. You can verify the status by using the AWS EC2 describe-instances command.
To stop multiple EC2 instances using the AWS CLI in a single command, you can provide the instance IDs for all the instances you want to stop. The AWS EC2 stop-instances command supports specifying multiple instance IDs.
Here’s an example:
aws ec2 stop-instances –instance-ids i-instance1 i-instance2 i-instance3
Replace i-instance1, i-instance2, and i-instance3 with the actual instance IDs of the EC2 instances you want to stop.
Reboot an EC2 Instance:
To reboot an EC2 instance using the AWS CLI, you can use the AWS EC2 reboot-instances command. Below is the basic command:
aws ec2 reboot-instances –instance-ids YourInstanceId
Replace YourInstanceId with the actual ID of the EC2 instance you want to reboot.
Here’s an example:
aws ec2 reboot-instances –instance-ids i-0abcdef1234567890
If successful, the command will output information about the instance state change. You can verify the status by using the AWS EC2 describe-instances command.
Terminate an EC2 Instance:
To terminate an EC2 instance using the AWS CLI, you can use the AWS EC2 terminate-instances command. Here’s the basic syntax:
aws ec2 terminate-instances –instance-ids YourInstanceId
Replace YourInstanceId with the actual ID of the EC2 instance you want to terminate.
Here’s an example:
aws ec2 terminate-instances –instance-ids i-1234567890abcdef0
Create a Key Pair:
To create an EC2 key pair using the AWS CLI, you can use the AWS EC2 create-key-pair command. Here’s the basic syntax:
aws ec2 create-key-pair –key-name YourKeyPairName –query ‘KeyMaterial’ –output text > YourKeyPair.pem
Replace YourKeyPairName with the desired name for your key pair. The –query ‘KeyMaterial’ –output text part of the command is used to extract and save the private key material to a local file (YourKeyPair.pem in this example).
Here’s an example:
aws ec2 create-key-pair –key-name DevKeyPair –query ‘KeyMaterial’ –output text > DevKeyPair.pem
List EC2 Security Groups:
To list security groups using the AWS CLI, you can use the AWS EC2 describe-security-groups command. Here’s the basic syntax:
aws ec2 describe-security-groups
This command will provide detailed information about all the security groups in your AWS account.
If you want to filter the output or display specific information about the security groups, you can use the –query parameter. For example, to display only the security group names and IDs in a table format, you can use:
aws ec2 describe-security-groups –query ‘SecurityGroups[*].[GroupId, GroupName]’ –output table
Adjust the –query parameter based on the specific information you are interested in.
Create a Security Group:
To create an EC2 security group using the AWS CLI, you can use the AWS EC2 create-security-group command. Below is the basic syntax:
aws ec2 create-security-group –group-name YourSecurityGroupName –description “YourSecurityGroupDescription”
Replace YourSecurityGroupName with the desired name for your security group and provide a meaningful description for YourSecurityGroupDescription.
Here’s an example:
aws ec2 create-security-group –group-name DevSecurityGroup –description “Dev security group”
This command will create a new security group named “DevSecurityGroup” with the specified description.
Authorize Ingress Rule in a Security Group:
After creating the security group, you may want to add inbound rules to control the traffic. You can use the authorize-security-group-ingress and authorize-security-group-egress commands for this purpose.
For example, to allow incoming SSH traffic (port 22) from a specific IP range:
aws ec2 authorize-security-group-ingress –group-name DevSecurityGroup –protocol tcp –port 22 –cidr 10.10.10.6/32
Replace the IP Address range with your required IP address.
Add Egress rule in a Security Group:
To add an egress (outbound) rule to an EC2 security group using the AWS CLI, you can use the authorize-security-group-egress command. Here’s the basic syntax:
aws ec2 authorize-security-group-egress –group-id YourSecurityGroupId –protocol YourProtocol –port YourPort –cidr YourDestination
Replace the placeholders with your specific values:
YourSecurityGroupId: The ID of your security group.
YourProtocol: The protocol for the rule (e.g., tcp, udp, icmp).
YourPort: The port number or range of ports (e.g., 80, 0-65535).
YourDestination: The destination IP address range or CIDR block.
Here’s an example command that allows all outbound traffic (0.0.0.0/0) on all ports:
aws ec2 authorize-security-group-egress –group-id sg-0123456789abcdef0 –protocol tcp –cidr 0.0.0.0/0
This command allows all outbound traffic from the specified security group (sg-0123456789abcdef0). Adjust the parameters based on your specific requirements.
Create an Amazon Machine Image (AMI):
To create an Amazon Machine Image (AMI) for an EC2 instance using the AWS CLI, you can use the AWS EC2 create-image command. Below is the basic syntax:
aws ec2 create-image –instance-id YourInstanceId –name “YourAMIName” –description “YourAMIDescription”
Replace the placeholders with your specific values:
YourInstanceId: The ID of the EC2 instance for which you want to create the AMI.
YourAMIName: A name for the new AMI.
YourAMIDescription: A description for the new AMI.
Here’s an example:
aws ec2 create-image –instance-id i-0abcdef1234567890 –name “Dev-Server-AMI” –description “AMI for Dev server”
This command will initiate the creation of an AMI for the specified EC2 instance. Note that this process may take some time.
After creating the AMI, you can use the resulting AMI ID to launch new instances with the same configuration as the original instance.
List EC2 AMIs:
To list EC2 Amazon Machine Images (AMIs) using the AWS CLI, you can use the AWS EC2 describe-images command. Here’s the basic syntax:
aws ec2 describe-images –owners self
This command lists the AMIs owned by the account associated with your AWS credentials. The –owners self parameter filters the results to show only the AMIs owned by the account.
If you want to list all AMIs, regardless of ownership, you can omit the –owners parameter:
aws ec2 describe-images
You can also filter the output using the –query parameter to display specific information. For example, to display only the AMI IDs and names in a table format:
aws ec2 describe-images –query ‘Images[*].[ImageId,Name]’ –output table
Conclusion
In conclusion, AWS CLI commands for Amazon Elastic Compute Cloud (EC2) provide a powerful and flexible way to manage your virtual server instances in the AWS environment. Ensure your AWS CLI is configured with the correct credentials. Exercise caution with commands that can modify or delete resources. By mastering these AWS CLI commands, you can efficiently manage your EC2 instances, security groups, and AMIs, providing a foundation for effective cloud infrastructure management.
Follow our Twitter and Facebook feeds for new releases, updates, insightful posts and more.